I have brushed up the 5 year old solution Poll for SharePoint, to change the deprecated “Google Image Charts to use Google Charts (AKA Google Visualization). The Image Charts are officially deprecated, but will probably continue working for some time, but to ensure you have a working solution, you should upgrade to this new version.
Please note that there are some changes to the Content Editor Web Part code, so existing users must not only update the script file, but also look over the CEWP code and make some small changes.
This code lets you generate polls without the need for server side installed WebParts.
Change log
March 15, 2015
v2.0 released. This one has no new functionality, but the code has been brushed up, and now the charts are generated using “Google Charts” rather than “Image Charts”.
Poll
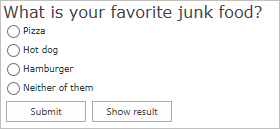
Result with column chart
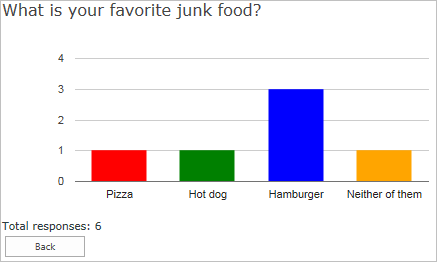
How to set it up
Create a custom list with the following fields
- Answer: Single line of text
- Question: Single line of text
Name it anything you like, but keep the display name fairly simple (no special characters) as you will use the display name in the CEWP code.
CEWP code
The CEWP code below refers jQuery from Google. If you have a local copy of jQuery you can change the script src. You find the code for the file “SPJS-Poll.js” at the bottom of the page.
NOTE: You must change the script src for the file “SPJS-Poll.js” and “spjs-utility.js” to point your instance of the files – the CEWP code will not work unless you do this.
Place this code where you want the poll to appear:
<div id="SPJS_Poll"></div>
<link type="text/css" href="/Scripts/Poll/spjs_poll.css" rel="stylesheet">
<script type="text/javascript" src="https://www.google.com/jsapi"></script>
<script type="text/javascript" src="https://code.jquery.com/jquery-1.11.2.min.js"></script>
<script type="text/javascript" src="/Scripts/spjs-utility/spjs-utility.js"></script>
<script type="text/javascript" src="/Scripts/Poll/SPJS-Poll.js"></script>
<script type="text/javascript">
// Preload the chart solution
google.load("visualization", "1", {"packages":["corechart","table"]});
// If you want to override these texts, uncomment the object and localize as you like.
/*
spjs.poll.text = {
"submitLinkText":"Submit",
"backLinkText":"Back",
"showResultBtnText":"Show result",
"pollNotActiveText":"The poll is not active prior to {0}",
"pollEndedText":"The poll ended on {0}",
"alreadyRespondedText":"You answered: ",
"totalResponsesLabel":"Total responses: ",
"chartLegendLabels":["Answer","Count"]
};
*/
spjs.poll.init({pollAnswerListName:'Poll',
listBaseUrl:L_Menu_BaseUrl,
id:"Poll_3", // Allowed characters id a-z, 0-9 - and _
start:"01/02/2015", // format: mm/dd/yyyy
end:"03/15/2015", // format: mm/dd/yyyy
singleResponse:false,
q:"What is your favorite junk food?",
qStyle:"font-size:1.5em;color:#444;",
aStyle:"font-size:xx-small",
a:["Pizza","Hot dog","Hamburger","Neither of them"], // Leave empty for free input
color:["red","green","blue","orange"],
forceLowerCaseAnswer:false, // Group result by lowercase
chart:"col", // table, bar, col or pie
height:200,
width:500});
</script>
Object attributes explained
- pollAnswerListName: DisplayName or GUID of the list that stores the answers
- listBaseUrl: The baseUrl of the site. This is like “/sites/hr” when the list is located in the site “hr” under “/sites”. Use L_Menu_BaseUrl (or omit the property) for current site.
- id: The unique id of the poll. All poll answers are stored in a list and this id is used to separate each poll
- start: Start date in the format mm/dd/yyyy
- end: End date in the format mm/dd/yyyy
- singleResponse: true for one reply per user, false for unlimited number of replies
- q: Poll question. To have a linefeed in the question, use <br>
- qStyle: CSS syntax style
- aStyle: CSS syntax style
- a: Answers in an array format. To use free input and not predefined answers, leave the array empty.
- color: Colors for the chart in an array format. This must have the same length as the previous parameter – one color for each answer
- forceLowerCaseAnswer: Primarily for use with free input to avoid getting two “series” when the only difference are uppercase characters.
- chart: “bar” for bar chart, “col” for column chart, “pie” for pie chart or “table” for a plain table.
- height: Height in pixels
- width: Width in pixels
Regarding free input
If you leave the attribute “a” as an empty array, the user can supply free text as “answer”. When using free input, the result are automatically presented as a table.
Download code
The code for the file SPJS-Poll.js. You find spjs-utility.js here.
Questions or feedback
Post any questions in the forum
Alexander
Like this:
Like Loading...