I have previously posted a JavaScript solution for approving multiple documents or list items. This is an updated version that adds two buttons in the ribbon: one for “Approve” and one for “Reject”. Refer the original solution for details, but please note that the variables for controlling the text values have changed.
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> <script type="text/javascript" src="/Scripts/ApproveOrRejectSelected.js"></script>
If you are happy with the default, English text, you can skip this next step.
To override the default text, add this to the CEWP below the script reference to “ApproveOrRejectSelected.js”,and change the text as you like:
<script type="text/javascript"> spjs.contentApproval.text = { "approveBtn":"Approve selected", "rejectBtn":"Reject selected", "groupLabel":"Approve or Reject", "working":"Processing items...", "done":"{0} items processed" }; </script>
You can wrap it like this to switch based on the logged in users language (in MUI environments):
switch(_spPageContextInfo.currentLanguage){ case 1044: // Add Norwegian values spjs.contentApproval.text = { "approveBtn":"Approve selected", "rejectBtn":"Reject selected", "groupLabel":"Approve or Reject", "working":"Processing items...", "done":"{0} items processed" }; break; case 1031: // Add German values spjs.contentApproval.text = { "approveBtn":"Approve selected", "rejectBtn":"Reject selected", "groupLabel":"Approve or Reject", "working":"Processing items...", "done":"{0} items processed" }; break; }
Alexander
Alexander,
Can this be repurposed to use some individual buttons on the page rather than using button on the ribbon? I’m am new to Javascript.
Yes, use this for approve:
and this for reject:
You need to add the script file “ApproveOrRejectSelected.js” to the page to be able to use these functions.
Alexander
Hi Alexander,
I tried them and it worked great; then I did something that caused it to stop working permanently. I went and changed the column type to a single line of text and then it broke. I have tried to create a new column name and it doesn’t appear to work. I only see a message that says processing items. I also try to put the command in the console and receive no errors. Do you have any idea what could be going on?
Side note, I have modified the code from the ‘_ModerationStatus’ to ‘_BEStatus’ which is my status column and it is a choice type of column.
Thanks,
Eduardo.
An additional note; I started the debugger in the IE Dev tools and I recieve the following message:
“Unable to get value of the property ‘toString’: object is null or undefined”
My guess is that your _BEStatus field is empty (null), and the script does not check this as the “__ModerationStatus” field would never be empty.
Go into the code and add a check to see if the value is null – you should see the line number in the dev console.
Alexander
Hi Alexander,
I was able to figure out the problem, it appears that if you remove the ‘Title with menu’ column from the view the script will just stay frozen on processing item.
Thanks,
Eduardo.
Hi Alex,
God Morning!
I need your help!. i think you may know noteboard webpart in sharepoint 2013.it is used for article pages to post the comments.
now my requirement is to retrive the comments in the page.
Is it possible to retrive the noteboard webpart comments from the page using javscript/jquery/REST. becuase these comments are stored in contentDB
Sorry, I’m not familiar with the noteboard webpart.
Alexander
Great solution! I noticed when you display items in batches, items approved in the first batch will cause the page to refresh. If the user go to the second batch the process runs, but the page does not refresh. Is there a way to force it refresh, so the user will see the changes?
Hi,
I don’t have this solution installed at the moment so I cannot check it, but I would suspect that the issue is caused by a # in the url preventing the refresh of the page – change this part of the code:
Alexander
Thanks Alex! That worked perfectly! I appreciate the help.
Greetings Alex,
Can we make the two individual button with colors ? Approve with Green Code , and Red code for Reject ?
Appreciated your always help.
I don’t have this solution in use anywhere and haven’t really looked at it since 2015, but I took a look at the js and you should be able to add some styling to the buttons if you look at the code in the file ApproveSelected.js.
Just locate the approve or reject button code and experiment by adding this to the a-tag or the span-tag of the approve button:
Alexander
thank you Alex for your reply. However, i’m not familiar with coding and i don’t have SP designer. what is the suatblbe script that should i add in the web part for two button ( Approve ) – Reject using this code , since i add it but not working
You must open ApproveSelected.js in any text editor like Notepad or Visual Studio Code and make these changes:
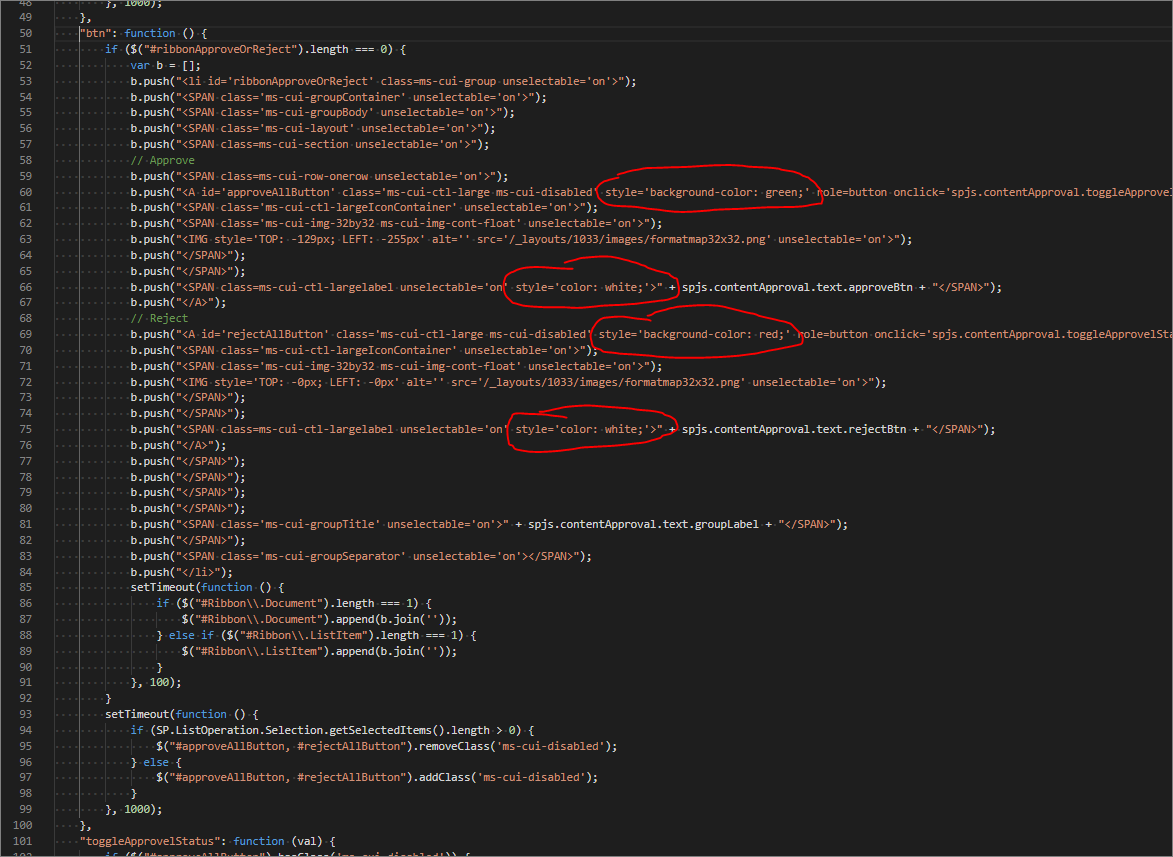
Alexander
Thank you Alex I changed what you wrote. Please have a look on it and feed back if its okay to be added in web part editor.
Thanks.
var spjs = spjs || {};
spjs.contentApproval = {
“localize”:function(){
if(spjs.contentApproval.text === undefined){
spjs.contentApproval.text = {
“approveBtn”:”Approve selected”,
“rejectBtn”:”Reject selected”,
“groupLabel”:”Approve or Reject”,
“working”:”Processing items…”,
“done”:”{0} items processed”
};
}
},
“data”:{
“notifyTimeout”:0,
“approvedOrRejectedCounter”:0,
“notifyId”:””
},
“init”:function(){
spjs.contentApproval.localize();
setTimeout(function(){
var defaultWP = document.getElementById(“MSOZoneCell_WebPartWPQ1”);
WpClick({target:defaultWP,srcElement:defaultWP});
},100);
setInterval(function(){
if($(“#RibbonContainer_activeTabId”).val()===’Ribbon.Document’ || $(“#RibbonContainer_activeTabId”).val()===’Ribbon.ListItem’){
spjs.contentApproval.btn();
}
},1000);
},
“btn”:function(){
if($(“#ribbonApproveOrReject”).length===0){
var b = [];
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
// Approve
b.push(“”);
b.push(““);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“” +spjs.contentApproval.text.approveBtn+””);
b.push(““);
// Reject
b.push(““);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“” +spjs.contentApproval.text.rejectBtn+””);
b.push(““);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”);
b.push(“”+spjs.contentApproval.text.groupLabel+””);
b.push(“”);
b.push(“”);
b.push(“”);
setTimeout(function(){
if($(“#Ribbon\\.Document”).length===1){
$(“#Ribbon\\.Document”).append(b.join(”));
}else if($(“#Ribbon\\.ListItem”).length===1){
$(“#Ribbon\\.ListItem”).append(b.join(”));
}
},100);
}
setTimeout(function(){
if(SP.ListOperation.Selection.getSelectedItems().length>0){
$(“#approveAllButton, #rejectAllButton”).removeClass(‘ms-cui-disabled’);
}else{
$(“#approveAllButton, #rejectAllButton”).addClass(‘ms-cui-disabled’);
}
},1000);
},
“toggleApprovelStatus”:function(val){
if($(“#approveAllButton”).hasClass(‘ms-cui-disabled’)){
return;
}
spjs.contentApproval.data.approvedOrRejectedCounter = 0;
spjs.contentApproval.data.notifyId = SP.UI.Notify.addNotification(spjs.contentApproval.text.working, true);
var cc, web, currLib, selectedItems, index;
cc = new SP.ClientContext.get_current();
web = cc.get_web();
cc.load(web);
currLib = web.get_lists().getById(SP.ListOperation.Selection.getSelectedList());
selectedItems = SP.ListOperation.Selection.getSelectedItems();
if(selectedItems.length>0){
for(index in selectedItems){
if(selectedItems[index].fsObjType === ‘0’){
spjs.contentApproval.checkAndUpdate(cc,currLib,selectedItems[index].id,val);
}
}
}
},
“notifyMe”:function(){
clearTimeout(spjs.contentApproval.data.notifyTimeout);
spjs.contentApproval.data.notifyTimeout = setTimeout(function(){
SP.UI.Notify.removeNotification(spjs.contentApproval.data.notifyId);
spjs.contentApproval.data.notifyId = SP.UI.Notify.addNotification(spjs.contentApproval.text.done.replace(/\{0\}/,spjs.contentApproval.data.approvedOrRejectedCounter), true);
setTimeout(function(){
SP.UI.Notify.removeNotification(spjs.contentApproval.data.notifyId);
if(spjs.contentApproval.data.approvedOrRejectedCounter>0){
location.href = location.href;
}
},1500);
},1000);
},
“checkAndUpdate”:function(cc,currLib,id,val){
var qb, camlQuery, collListItem;
qb = [];
qb.push(“”);
qb.push(“”+id+””);
qb.push(“”);
camlQuery = new SP.CamlQuery();
camlQuery.set_viewXml(qb.join(”));
collListItem = currLib.getItems(camlQuery);
cc.load(collListItem, “Include(ID,_ModerationStatus)”);
cc.executeQueryAsync(
function(sender, args){
var listEnumerator, curr, status;
listEnumerator = collListItem.getEnumerator();
while(listEnumerator.moveNext()){
curr = listEnumerator.get_current();
status = curr.get_item(‘_ModerationStatus’);
if(status !== val){
spjs.contentApproval.data.approvedOrRejectedCounter ++;
curr.set_item(‘_ModerationStatus’,val);
curr.update();
cc.executeQueryAsync(
function(sender, args){
//notifyMe();
},
function (sender, args) {
alert(args.get_message());
}
);
}
}
spjs.contentApproval.notifyMe();
},
function(sender, args){
alert(“Error:\n”+args.get_message());
}
);
}
};
ExecuteOrDelayUntilScriptLoaded(spjs.contentApproval.init, “sp.ribbon.js”);
The code must be added like described here to work: https://spjsblog.com/2010/01/08/how-to-post-code-in-comments/
Here is how it should look in your code:
Thank you very much Alex , i will do and ‘ll feed you back.
Greetings Alex,
i’ve added the JS file to the SiteAsset, however what should i add in the content editor or Snippet to call this file.
Thanks.
This snippet is the full content of the file ApproveOrRejectSelected.js – read the instructions in the top of the article and set it up like described.
Alexander